Bencode to JSON Converter in Rust
We're excited to introduce bencode2json, a crate that simplifies converting Bencode data to JSON, benefiting the Rust BitTorrent community.
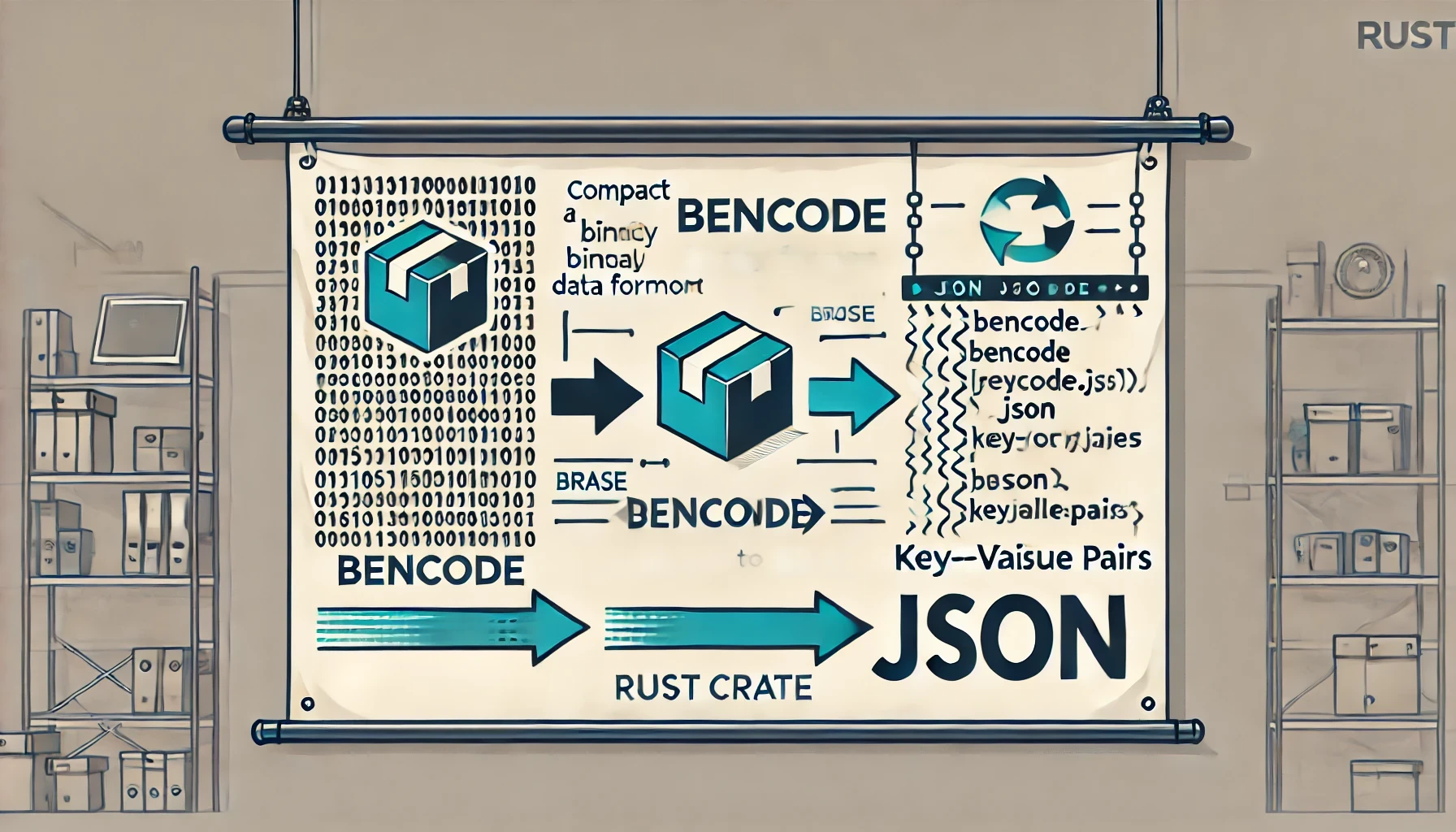
Table of contents
Introduction
Hello Bittorrent-in-Rust Community!
At Torrust, we’re committed to enhancing the BitTorrent ecosystem within the Rust community. Today, we’re excited to introduce a Bencode to JSON converter:
It’s a new crate designed to simplify the conversion of Bencode data into JSON format. This tool not only supports our Tracker but also aims to benefit the wider Rust community involved in BitTorrent projects.
Here’s a quick example to show how straightforward it is to use:
echo "4:spam" | cargo run
"spam"
For non UTF-8 Bencoded strings, we represent them as byte sequences enclosed in HTML-styled tags <hex>...</hex>
;
printf "d3:bar2:ÿþe" | cargo run
{"bar":"<hex>fffe</hex>"}
Why It Is Useful
Bencode is the encoding format used by BitTorrent, while JSON is a widely-used data interchange format. Converting Bencode to JSON can facilitate easier data manipulation, debugging, and integration with modern web applications. Here are a few reasons why bencode2json
is valuable:
- Interoperability: JSON is supported by most programming languages and libraries, making it easier to work with Bencoded data in diverse environments.
- Readability: JSON is often easier to read and understand compared to Bencode, especially for developers unfamiliar with the latter.
- Integration: Many web services and APIs utilize JSON, so having Bencode data readily available in this format can simplify integrations and data exchanges.
How You Can Use It
Using bencode2json
is straightforward. Here’s a quick guide on how to get started:
Installation
Add the crate to your Cargo.toml
:
[dependencies]
bencode2json = "0.1.0"
Basic Usage
Here’s a simple example of how to convert Bencode to JSON:
use bencode2json::try_bencode_to_json;
fn main() {
use torrust_bencode2json::{try_bencode_to_json};
let result = try_bencode_to_json(b"d4:spam4:eggse").unwrap();
assert_eq!(result, r#"{"spam":"eggs"}"#);
}
Advance Usage
bencode2json
supports any kind of input and output that implement Read or Write traits,
meaning you can read from stdin and write to stdout or from/to files.
use bencode2json::parsers::{BencodeParser};
let mut output = String::new();
let mut parser = BencodeParser::new(&b"4:spam"[..]);
parser
.write_str(&mut output)
.expect("Bencode to JSON conversion failed");
println!("{output}"); // It prints the JSON string: "spam"
Using the Console App
The package provides a console command too. You can install it with:
cargo install torrust-bencode2json
And execute it to convert a torrent file into JSON.
cat ./sample.torrent | cargo run | jq
jq is jq is a lightweight and flexible command-line JSON processor. Make sure you have it installed.
Performance
The converter only generates temporary in-memory representations for strings, ensuring memory consumption is proportional to the size of the largest Bencoded string. This design makes bencode2json efficient for both small and large datasets.
How You Can Contribute
We’re excited to see how the community can help improve bencode2json. Here are some ways you can get involved:
- Feature Requests: If you have ideas for features or improvements, please open an issue on the GitHub repository.
- Code Contributions: Help us build an opposite converter, JSON to Bencode, by submitting a pull request. This could greatly enhance the functionality of the crate and provide a complete solution for working with these data formats.
- Documentation: Contributing to the documentation is always valuable. Clear examples and explanations help other users understand and utilize the crate effectively.
- Feedback: Share your experience using bencode2json. Your feedback will help us make it even better!
Conclusion
bencode2json
is a step forward in bridging the gap between Bencode and JSON within the BitTorrent ecosystem in Rust. We encourage everyone to try it out, contribute, and join us in fostering a collaborative environment for Rust developers. Happy coding!
Check out the bencode2json GitHub repository to get started and contribute to the project. Let’s make working with BitTorrent in Rust easier together!
Acknowledgments
This implementation is basically a port to Rust from https://gist.github.com/camilleoudot/840929699392b3d25afbec25d850c94a with some changes like:
- It does not use magic numbers (explicit enum for states).
- It prints non UTF-8 string in hexadecimal.
The idea of using hexadecimal format <hex>ff</hex>
for non UTF-8 string came from the bencode online repo by @Chocobo1.
We also want to thank @da2ce7 for his feedback and review that has improved this project significantly.
If you have any questions or issues regarding this post, please open an issue.
We very welcome any contributions to the project!